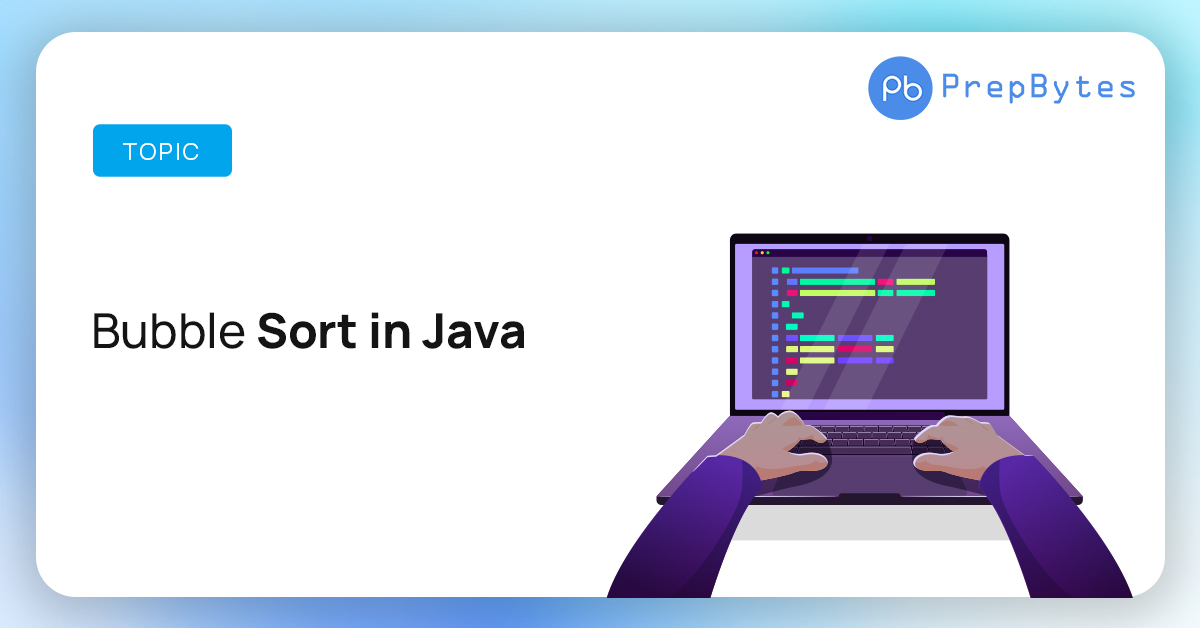
Bubble Sort in Java
Bubble sort is a simple algorithm that compares the first element of the array to the next one. If the current element of the array is numerically greater than the next one, the elements are swapped. Likewise, the algorithm will traverse the entire element of the array. In this tutorial, we will create a JAVA program to implement Bubble Sort.

Elegant Bubble Sort Java
Bubble Sort in Java We can create a java program to sort array elements using bubble sort. Bubble sort algorithm is known as the simplest sorting algorithm. In bubble sort algorithm, array is traversed from first element to last element. Here, current element is compared with the next element.
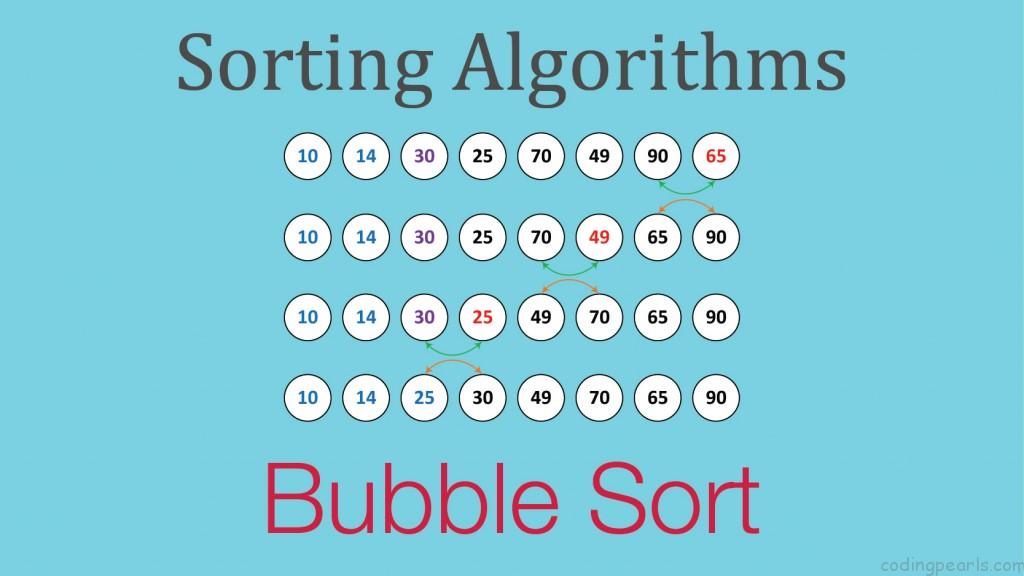
Bubble Sort trong Java Học Java
Bubble sort in Java is the simplest, comparison-based sorting algorithm which helps us sort an array by traversing it repeatedly, comparing adjacent elements, and swapping them based upon desired sorting criteria. Bubble sort is an in-place algorithm, meaning it does not require any extra space; it changes the original array instead.
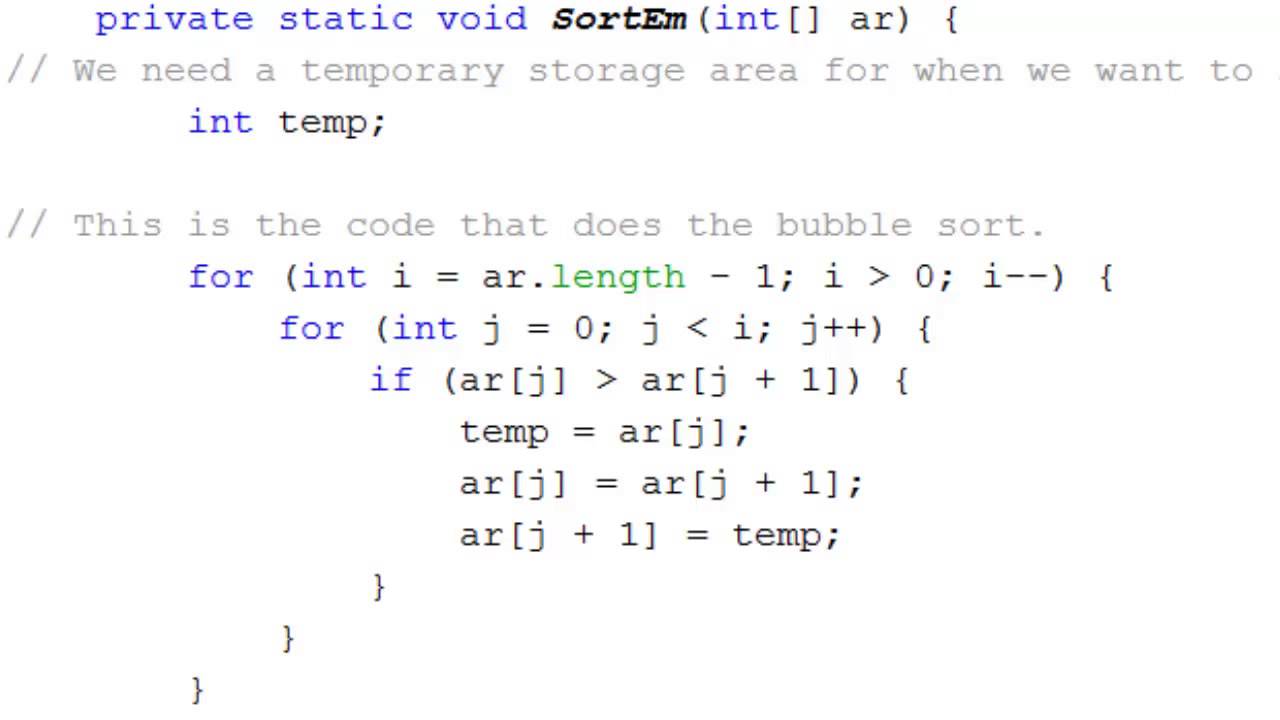
Java Bubble Sort YouTube
Bubble Sort Bubble sort is a sorting algorithm that compares two adjacent elements and swaps them until they are in the intended order. Just like the movement of air bubbles in the water that rise up to the surface, each element of the array move to the end in each iteration. Therefore, it is called a bubble sort. Working of Bubble Sort
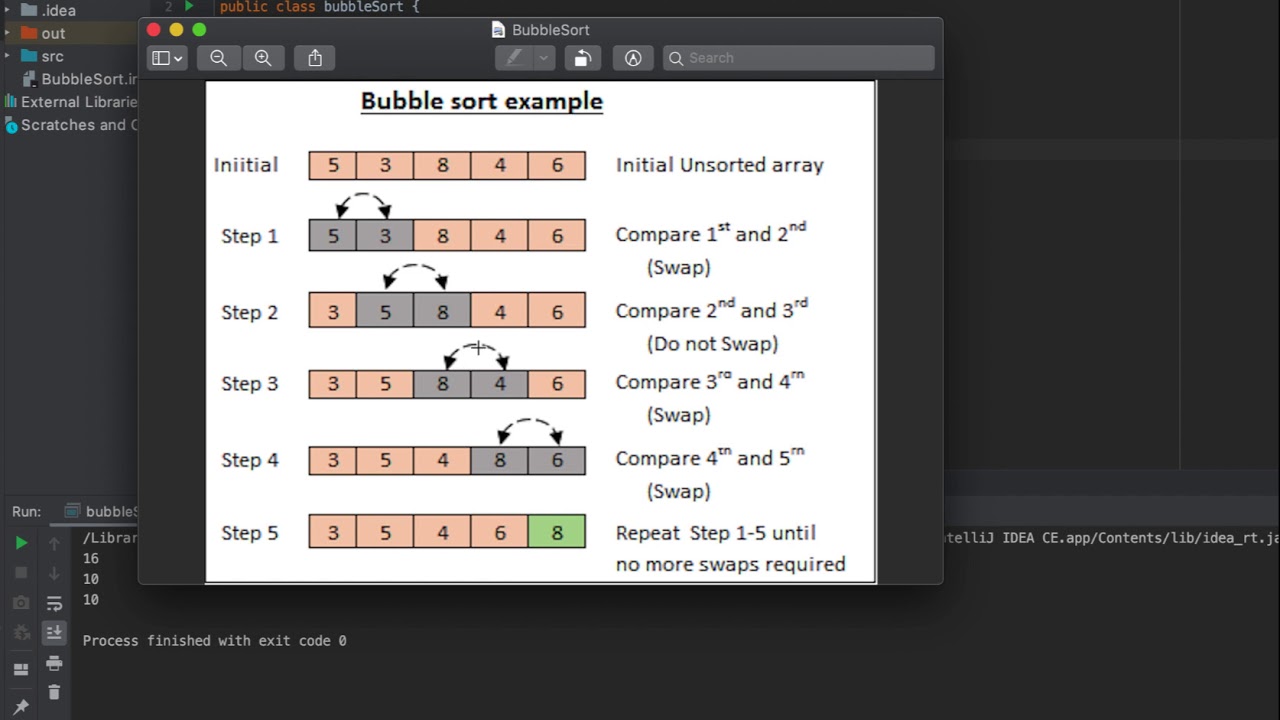
Efficient Bubble Sort in Java YouTube
Step 1: For i = 0 to N-1 repeat Step 2 Step 2: For J = i + 1 to N - I repeat Step 3: if A [J] > A [i] Swap A [J] and A [i]
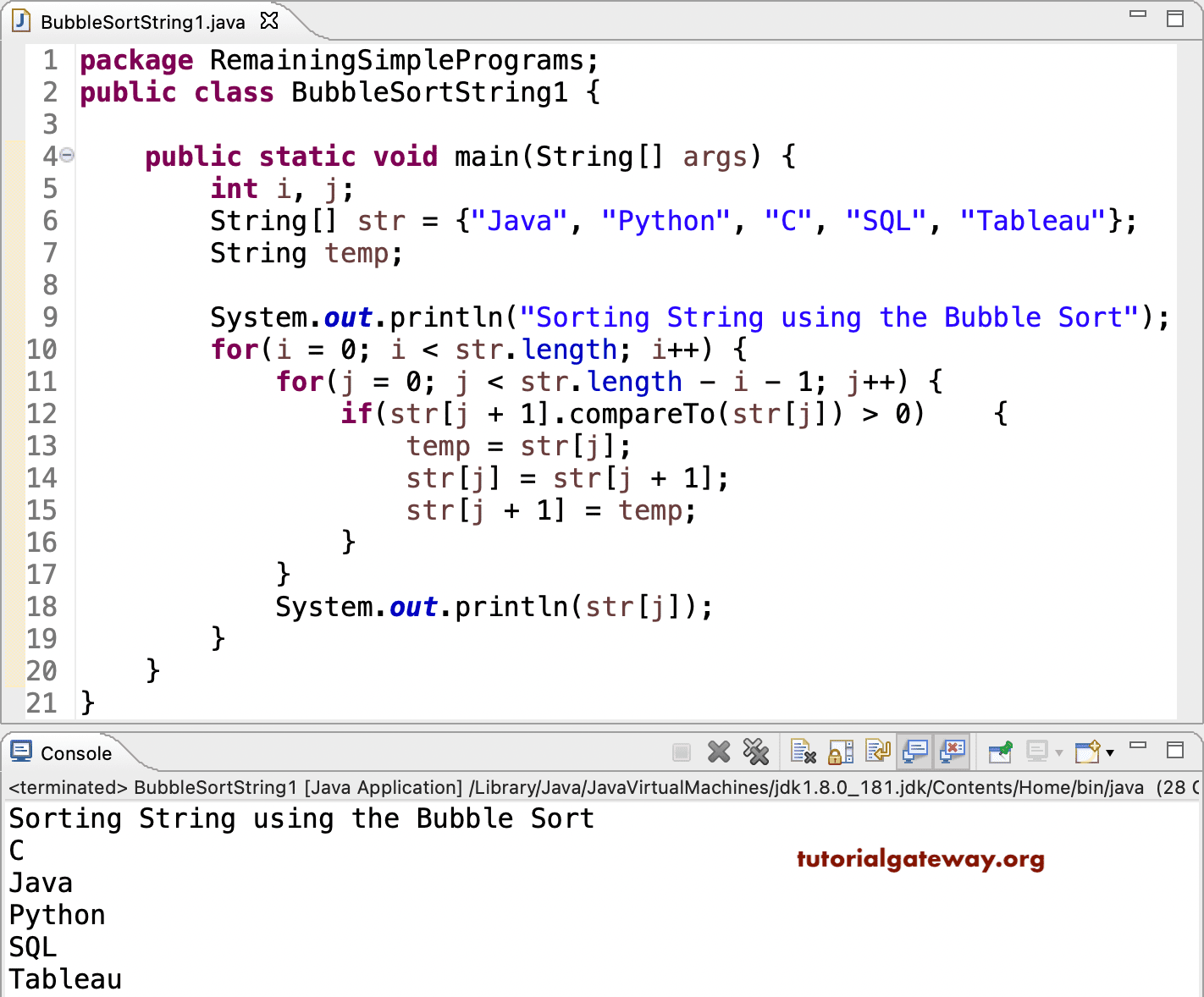
Java Program to Perform Bubble Sort on Strings
Output 1 Choose Sorting Order: 1 for Ascending 2 for Descending 1 Sorted Array: [-9, -2, 0, 11, 45] In this case, we have entered 1 as input. Hence, the program sort the array in ascending order. Output 2 Choose Sorting Order: 1 for Ascending 2 for Descending 2 Sorted Array: [45, 11, 0, -2, -9] In this case, we have entered 2 as input.
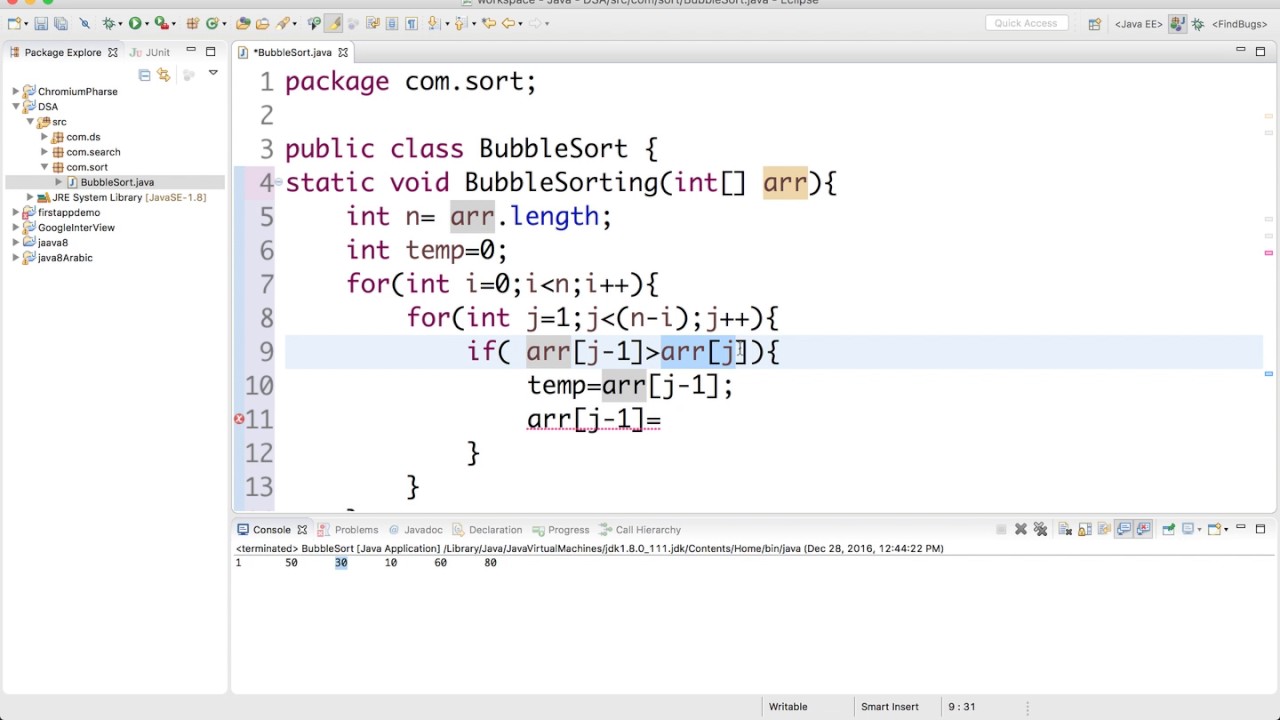
26 Bubble Sort implementaion in Java YouTube
It is called bubble sort because the movement of array elements is just like the movement of air bubbles in the water. Bubbles in water rise up to the surface; similarly, the array elements in bubble sort move to the end in each iteration.
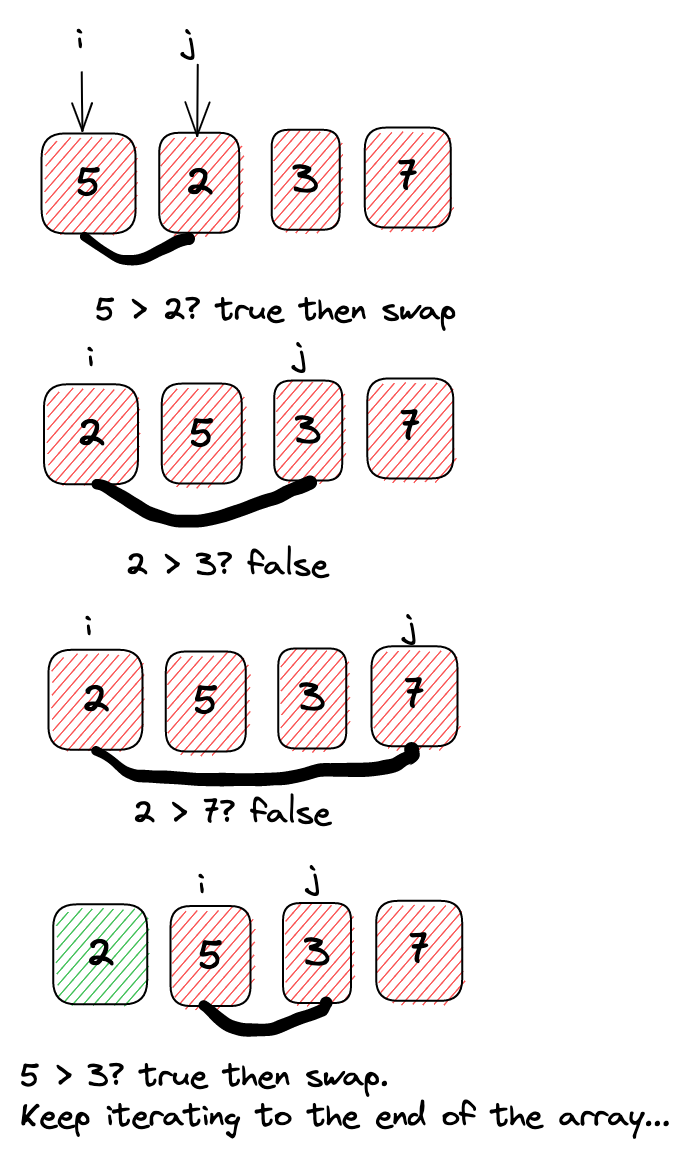
Bubble Sort with Java Java Challengers
Implementing bubble sort in Java involves creating a function that uses nested loops to compare and swap adjacent elements in an array. Here's a simple example:
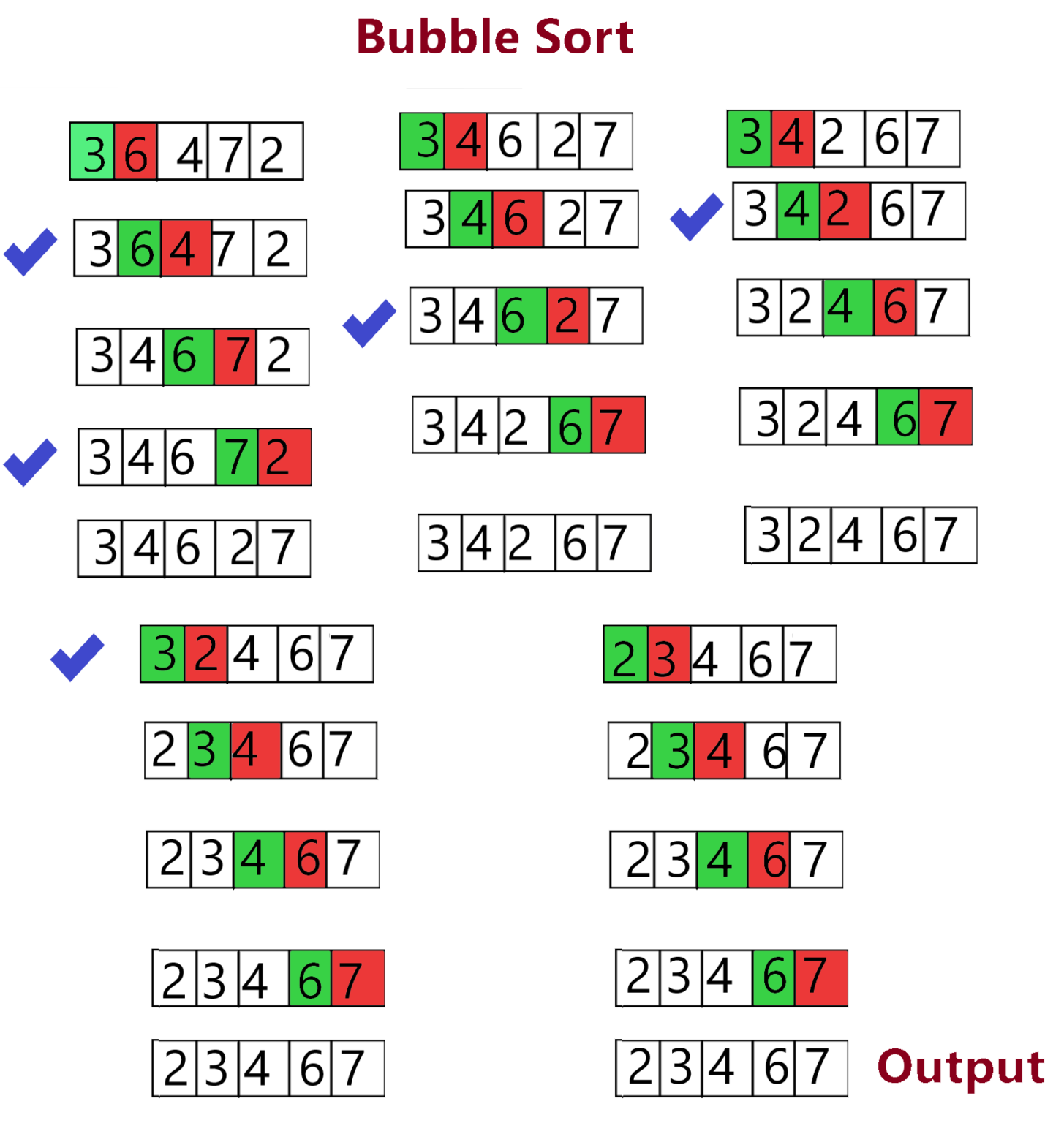
Bubble Sort Java Algorithm, Working, Examples TechBlogStation
Bubble Sort Algorithm (optimized) in Java. Here is the algorithm for bubble sort in Java: An outer loop says i, (journeys) from [0, n-1) ( n = length of the array). An inner loop say j, from [0, n - outer Loop count - 1). if element [j] > element [j+1] -> swap. Below is the complete code to implement Bubble Sort in Java:
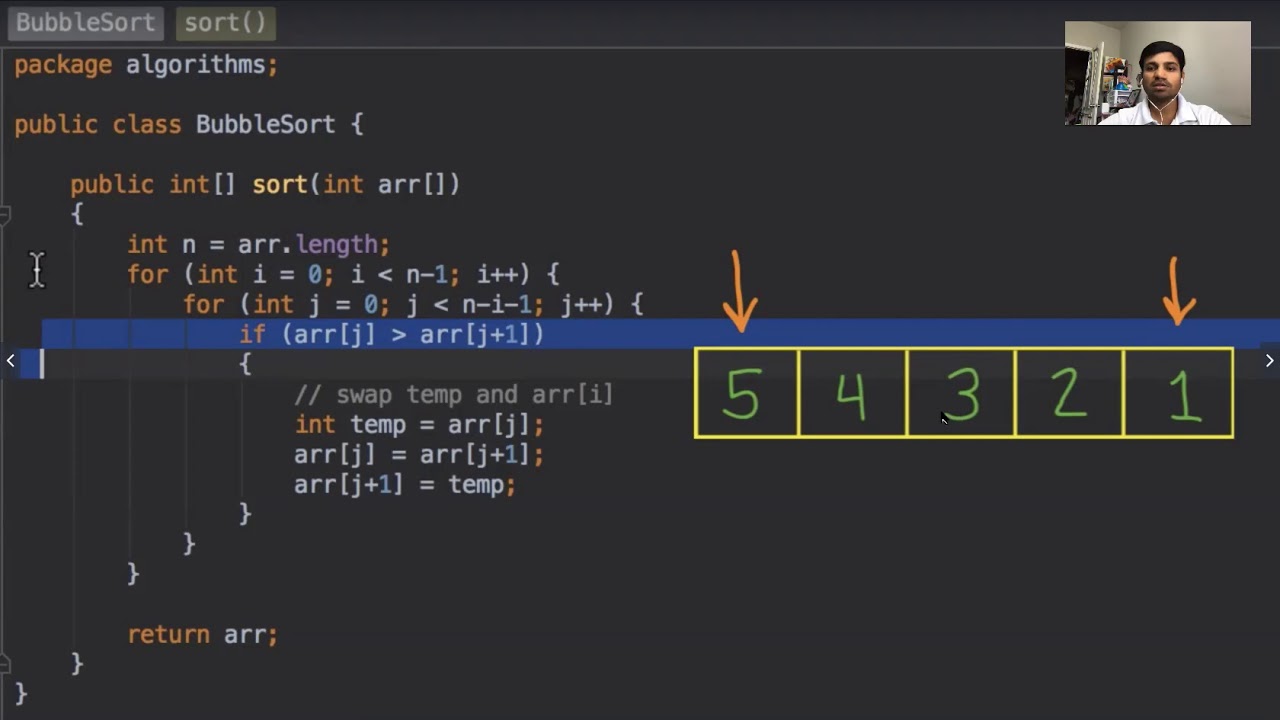
Bubble sort implementation with Test Driven approach in Java YouTube
2. Methodology As mentioned earlier, to sort an array, we iterate through it while comparing adjacent elements, and swapping them if necessary. For an array of size n, we perform n-1 such iterations. Let's take up an example to understand the methodology. We'd like to sort the array in the ascending order: 4 2 1 6 3 5
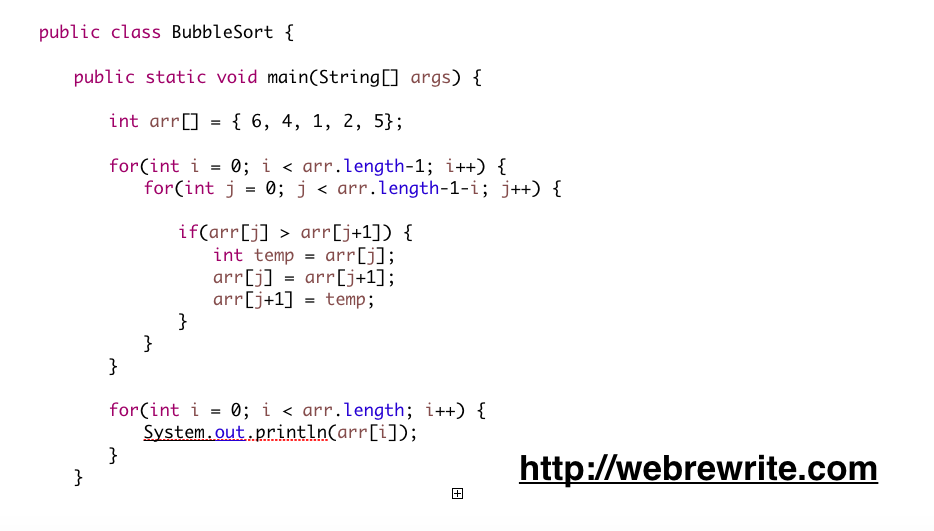
Bubble Sort Program in Java Explained through Video Tutorial
The bubble sort algorithm functions by repeatedly comparing and swapping adjacent elements of an array until the complete array is sorted. The term "bubble" in its name refers to the way in which an element shifts in the array, resembling the movement of air bubbles in the water.As water bubbles ascend to the surface, the elements in the array move toward the end with each iteration.
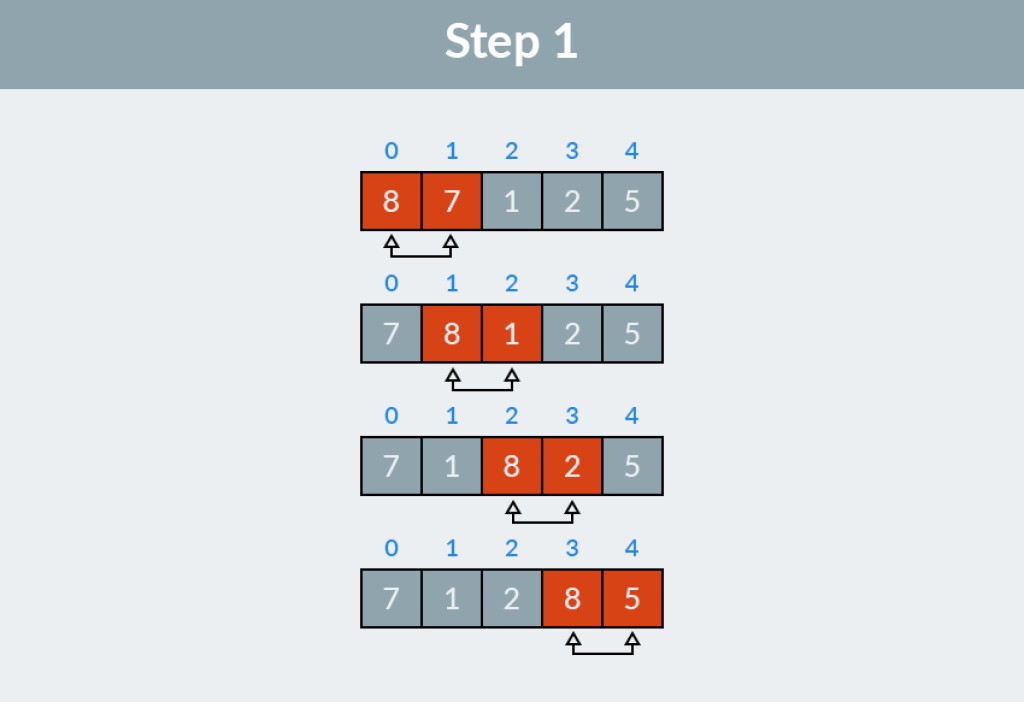
Bubble Sort Java
Bubble Sort Overview. Bubble sort is the simplest sorting algorithm. It works by iterating the input array from the first element to the last, comparing each pair of elements and swapping them if needed. Bubble sort continues its iterations until no more swaps are needed. This algorithm is not suitable for large datasets as its average and.

Bubble Sort Java Algorithm, Working, Examples TechBlogStation
Bubble sort Java code Bubble sort Java realisation Let's create two methods for Bubble sort. The first one, bubbleSort(int[] myArray) is a plane one. It runs through the array every time. The second one, optimizedBubbleSort(int myArray[]) is optimized by stopping the algorithm if inner loop didn't cause any swap. Counter shows you how many.
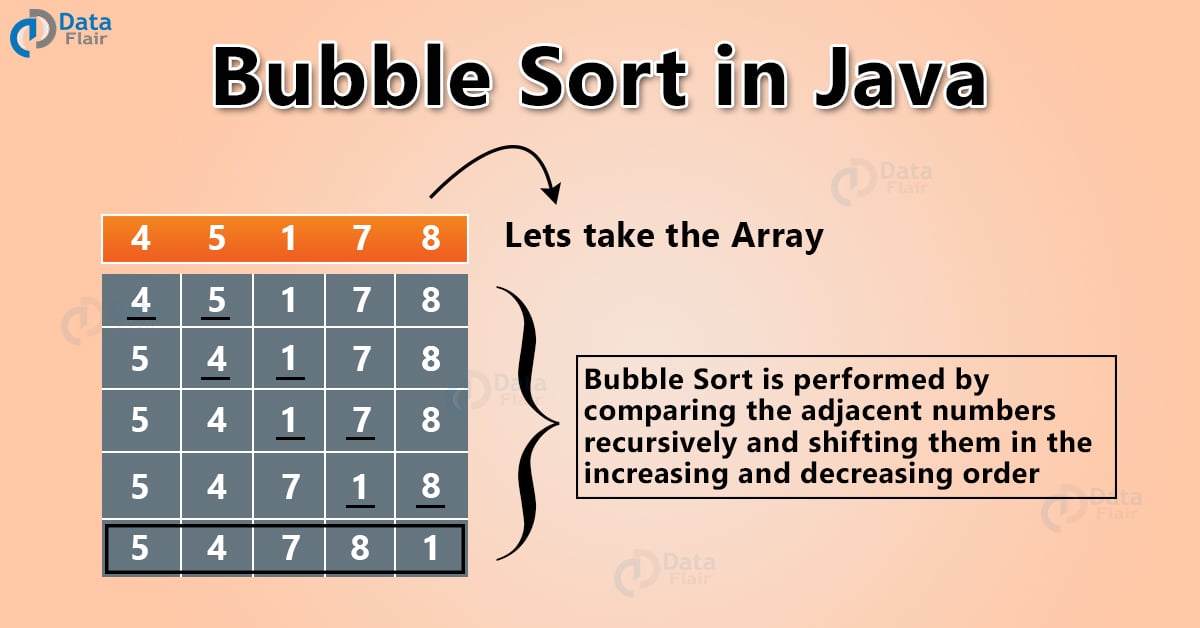
Bubble Sort in Java Learn How to Implement with Example! DataFlair
C++ Code Example of Bubble Sort Algorithm. Like I did for Java, I also added comments to the implementation of the bubble sort algorithm in C++ because it's more verbose than that of Python and Java: #include
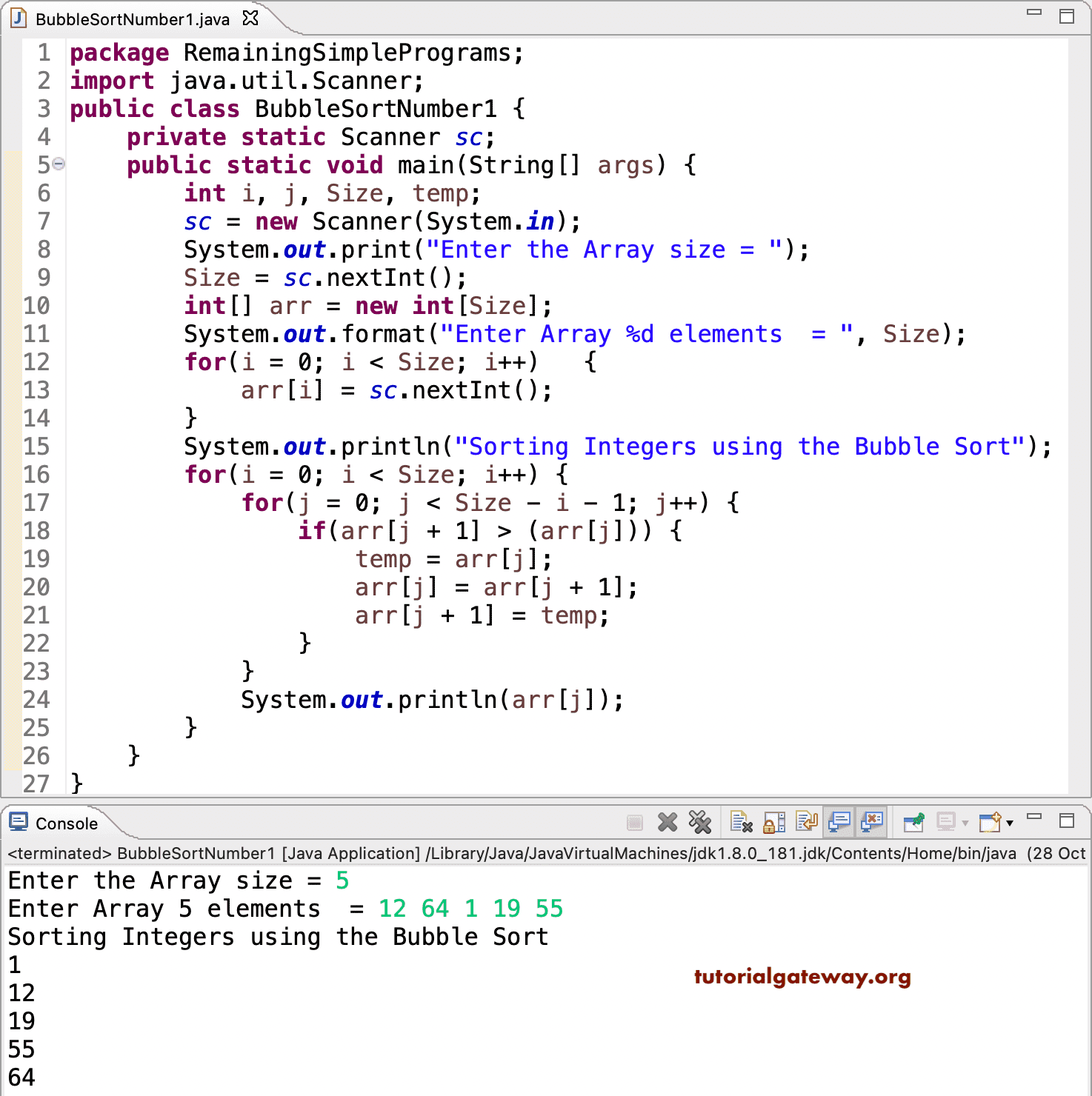
Java Bubble Sort Program on Integers
Sorting is a process of arranging items in a sequential order, based on some criterion. There are several algorithms that are used for sorting and one amongst them is Bubble sort. Bubble sort algorithm is known as the simplest sorting algorithm. So this article on Bubble Sort in Java will help you understand this concept in detail.
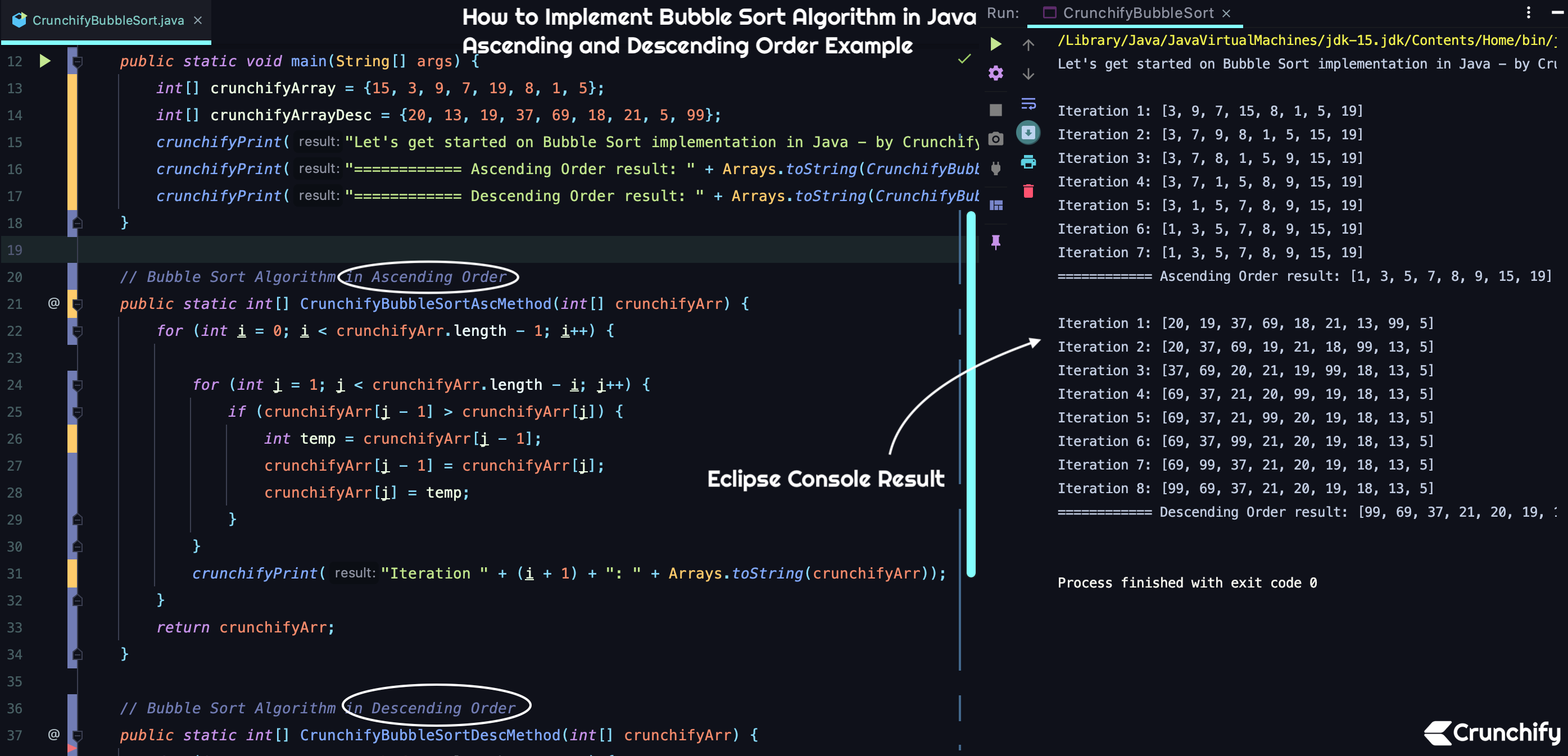
How to Implement Bubble Sort Algorithm in Java Ascending and Descending Order Example • Crunchify
Some important points about the Bubble sort are: 1. The Worst and Average case complexity of Bubble Sort is O(n2), where n denotes the total number of elements in the array.. 2. The Best case complexity of Bubble sort is O(n), where n is the number of elements in the array.This case can happen only when the given array is already sorted. 3. The Auxiliary space consumed by the bubble sort.